Laboratory 4
Chapter 14: GUI Components, Part 1
Before the lab, do the Self-Review exercises in the chapter.
Put the Java source code of your solution to the exercise below on your private GitHub repository:
Feel free to talk to whomever you like about any aspect of the laboratory. Working in pairs may be helpful.
Chapter 14
Make a Java application whose control panel looks like the screen shot below.
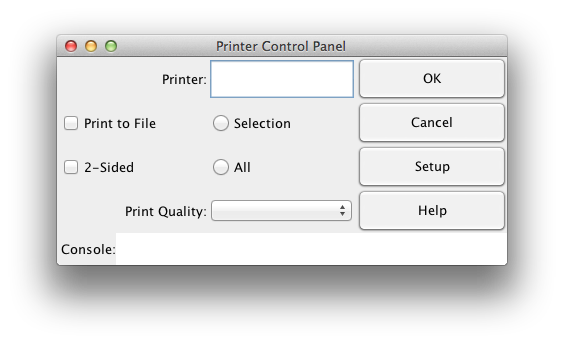
import java.awt.BorderLayout; import java.awt.Dimension; import javax.swing.JFrame; /** * * @author Pete Cappello */ public class App extends JFrame { private static final int HEIGHT = 230; private static final int WIDTH = 450; private final Controller controller; App() { controller = new Controller(); setTitle( "Printer Control Panel" ); setDefaultCloseOperation( JFrame.EXIT_ON_CLOSE ); add( controller, BorderLayout.CENTER ); Dimension dimension = new Dimension( WIDTH, HEIGHT ); setSize( dimension ); setPreferredSize( dimension ); setVisible( true ); } /** * Run the Graphics Virtual Machine application. * @param args unused */ public static void main( String[] args ) { App app = new App(); } }
Notes
- The filed to the right of the "Printer:" JLabel is a JTextField.
- The filed to the right of the "Printer Quality:" JLabel is a JComboBox. The filed to the right of the "Console:" JLabel is a JTextArea, intitalized to 2 rows & 80 columns.
- To get the layout above, I used, among other things, the JLabel.setHorizontalAlignment method. Please see JLabel's Javadoc.